Java AES加密和解密教程
在本教程中,我們將看到如何使用JDK中的Java密碼體系結構(JCA)來實現AES加密和解密。對稱密鑰塊密碼在數據加密中起重要作用。這意味著同一密鑰可用于加密和解密。高級加密標準(AES)是一種廣泛使用的對稱密鑰加密算法。
AES算法是一種迭代的對稱密鑰塊密碼,它支持128、192和256位的加密密鑰(秘密密鑰),以對128位的塊中的數據進行加密和解密。
在AES中生成密鑰的方法有兩種:從隨機數生成或從給定密碼生成。
在第一種方法中,應該從像SecureRandom類這樣的加密安全(偽)隨機數生成器生成秘密密鑰。為了生成密鑰,我們可以使用KeyGenerator類。讓我們定義一種用于生成大小為n(128、192和256)位的AES密鑰的方法:
public static SecretKey generateKey(int n) throws NoSuchAlgorithmException { KeyGenerator keyGenerator = KeyGenerator.getInstance('AES'); keyGenerator.init(n); SecretKey key = keyGenerator.generateKey(); return key;}
在第二種方法中,可以使用基于密碼的密鑰派生功能(例如PBKDF2)從給定的密碼派生AES秘密密鑰。下面方法可通過65,536次迭代和256位密鑰長度從給定密碼生成AES密鑰:
public static SecretKey getKeyFromPassword(String password, String salt) throws NoSuchAlgorithmException, InvalidKeySpecException {SecretKeyFactory factory = SecretKeyFactory.getInstance('PBKDF2WithHmacSHA256'); KeySpec spec = new PBEKeySpec(password.toCharArray(), salt.getBytes(), 65536, 256); SecretKey secret = new SecretKeySpec(factory.generateSecret(spec).getEncoded(), 'AES'); return secret;}
加密字符串
要實現輸入字符串加密,我們首先需要根據上一節生成密鑰和初始化向量IV:
IV是偽隨機值,其大小與加密的塊相同。我們可以使用SecureRandom類生成隨機IV。
讓我們定義一種生成IV的方法:
public static IvParameterSpec generateIv() { byte[] iv = new byte[16]; new SecureRandom().nextBytes(iv); return new IvParameterSpec(iv);}
下一步,我們使用getInstance()方法從Cipher類創建一個實例。
此外,我們使用帶有秘密密鑰,IV和加密模式的init()方法配置密碼實例。最后,我們通過調用doFinal()方法對輸入字符串進行加密。此方法獲取輸入字節并以字節為單位返回密文:
public static String encrypt(String algorithm, String input, SecretKey key, IvParameterSpec iv) throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException {Cipher cipher = Cipher.getInstance(algorithm); cipher.init(Cipher.ENCRYPT_MODE, key, iv); byte[] cipherText = cipher.doFinal(input.getBytes()); return Base64.getEncoder().encodeToString(cipherText);}
為了解密輸入字符串,我們可以使用DECRYPT_MODE初始化密碼來解密內容:
public static String decrypt(String algorithm, String cipherText, SecretKey key, IvParameterSpec iv) throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException {Cipher cipher = Cipher.getInstance(algorithm); cipher.init(Cipher.DECRYPT_MODE, key, iv); byte[] plainText = cipher.doFinal(Base64.getDecoder().decode(cipherText)); return new String(plainText);}
編寫一個用于加密和解密字符串輸入的測試方法:
@Testvoid givenString_whenEncrypt_thenSuccess() throws NoSuchAlgorithmException, IllegalBlockSizeException, InvalidKeyException, BadPaddingException, InvalidAlgorithmParameterException, NoSuchPaddingException { String input = 'baeldung'; SecretKey key = AESUtil.generateKey(128); IvParameterSpec ivParameterSpec = AESUtil.generateIv(); String algorithm = 'AES/CBC/PKCS5Padding'; String cipherText = AESUtil.encrypt(algorithm, input, key, ivParameterSpec); String plainText = AESUtil.decrypt(algorithm, cipherText, key, ivParameterSpec); Assertions.assertEquals(input, plainText);}
加密文件
現在,讓我們使用AES算法加密文件。步驟是相同的,但是我們需要一些IO類來處理文件。讓我們加密一個文本文件:
public static void encryptFile(String algorithm, SecretKey key, IvParameterSpec iv, File inputFile, File outputFile) throws IOException, NoSuchPaddingException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException {Cipher cipher = Cipher.getInstance(algorithm); cipher.init(Cipher.ENCRYPT_MODE, key, iv); FileInputStream inputStream = new FileInputStream(inputFile); FileOutputStream outputStream = new FileOutputStream(outputFile); byte[] buffer = new byte[64]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) {byte[] output = cipher.update(buffer, 0, bytesRead);if (output != null) { outputStream.write(output);} } byte[] outputBytes = cipher.doFinal(); if (outputBytes != null) {outputStream.write(outputBytes); } inputStream.close(); outputStream.close();}
請注意,不建議嘗試將整個文件(尤其是大文件)讀入內存。相反,我們一次加密一個緩沖區。
為了解密文件,我們使用類似的步驟,并使用DECRYPT_MODE初始化密碼,如前所述。
再次,讓我們定義一個用于加密和解密文本文件的測試方法。在這種方法中,我們從測試資源目錄中讀取baeldung.txt文件,將其加密為一個名為baeldung.encrypted的文件,然后將該文件解密為一個新文件:
@Testvoid givenFile_whenEncrypt_thenSuccess() throws NoSuchAlgorithmException, IOException, IllegalBlockSizeException, InvalidKeyException, BadPaddingException, InvalidAlgorithmParameterException, NoSuchPaddingException {SecretKey key = AESUtil.generateKey(128); String algorithm = 'AES/CBC/PKCS5Padding'; IvParameterSpec ivParameterSpec = AESUtil.generateIv(); Resource resource = new ClassPathResource('inputFile/baeldung.txt'); File inputFile = resource.getFile(); File encryptedFile = new File('classpath:baeldung.encrypted'); File decryptedFile = new File('document.decrypted'); AESUtil.encryptFile(algorithm, key, ivParameterSpec, inputFile, encryptedFile); AESUtil.decryptFile( algorithm, key, ivParameterSpec, encryptedFile, decryptedFile); assertThat(inputFile).hasSameTextualContentAs(decryptedFile);}
基于密碼加密解密
我們可以使用從給定密碼派生的密鑰進行AES加密和解密。
為了生成密鑰,我們使用getKeyFromPassword()方法。加密和解密步驟與字符串輸入部分中顯示的步驟相同。然后,我們可以使用實例化的密碼和提供的密鑰來執行加密。
讓我們寫一個測試方法:
@Testvoid givenPassword_whenEncrypt_thenSuccess() throws InvalidKeySpecException, NoSuchAlgorithmException, IllegalBlockSizeException, InvalidKeyException, BadPaddingException, InvalidAlgorithmParameterException, NoSuchPaddingException {String plainText = 'www.baeldung.com'; String password = 'baeldung'; String salt = '12345678'; IvParameterSpec ivParameterSpec = AESUtil.generateIv(); SecretKey key = AESUtil.getKeyFromPassword(password,salt); String cipherText = AESUtil.encryptPasswordBased(plainText, key, ivParameterSpec); String decryptedCipherText = AESUtil.decryptPasswordBased( cipherText, key, ivParameterSpec); Assertions.assertEquals(plainText, decryptedCipherText);}
加密對象
為了加密Java對象,我們需要使用SealedObject類。該對象應可序列化。讓我們從定義學生類開始:
public class Student implements Serializable { private String name; private int age; // standard setters and getters}
接下來,讓我們加密Student對象:
public static SealedObject encryptObject(String algorithm, Serializable object, SecretKey key, IvParameterSpec iv) throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, IOException, IllegalBlockSizeException {Cipher cipher = Cipher.getInstance(algorithm); cipher.init(Cipher.ENCRYPT_MODE, key, iv); SealedObject sealedObject = new SealedObject(object, cipher); return sealedObject;}
稍后可以使用正確的密碼解密加密的對象:
public static Serializable decryptObject(String algorithm, SealedObject sealedObject, SecretKey key, IvParameterSpec iv) throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, ClassNotFoundException, BadPaddingException, IllegalBlockSizeException, IOException {Cipher cipher = Cipher.getInstance(algorithm); cipher.init(Cipher.DECRYPT_MODE, key, iv); Serializable unsealObject = (Serializable) sealedObject.getObject(cipher); return unsealObject;}
讓我們寫一個測試用例:
@Testvoid givenObject_whenEncrypt_thenSuccess() throws NoSuchAlgorithmException, IllegalBlockSizeException, InvalidKeyException, InvalidAlgorithmParameterException, NoSuchPaddingException, IOException, BadPaddingException, ClassNotFoundException {Student student = new Student('Baeldung', 20); SecretKey key = AESUtil.generateKey(128); IvParameterSpec ivParameterSpec = AESUtil.generateIv(); String algorithm = 'AES/CBC/PKCS5Padding'; SealedObject sealedObject = AESUtil.encryptObject( algorithm, student, key, ivParameterSpec); Student object = (Student) AESUtil.decryptObject( algorithm, sealedObject, key, ivParameterSpec); assertThat(student).isEqualToComparingFieldByField(object);}
可以在GitHub上獲得本文的完整源代碼 。
以上就是Java AES加密和解密教程的詳細內容,更多關于Java AES加密和解密的資料請關注好吧啦網其它相關文章!
相關文章:
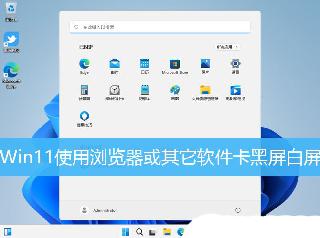