JAVA實現(xiàn)PDF轉(zhuǎn)HTML文檔的示例代碼
本文是基于PDF文檔轉(zhuǎn)PNG圖片,然后進行圖片拼接,拼接后的圖片轉(zhuǎn)為base64字符串,然后拼接html文檔寫入html文件實現(xiàn)PDF文檔轉(zhuǎn)HTML文檔。
引入Maven依賴
<!-- https://mvnrepository.com/artifact/org.apache.pdfbox/pdfbox --> <dependency><groupId>org.apache.pdfbox</groupId><artifactId>pdfbox</artifactId><version>2.0.12</version> </dependency>
工具實現(xiàn)類
package com.frame.utils;import org.apache.pdfbox.pdmodel.PDDocument;import org.apache.pdfbox.rendering.PDFRenderer;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import sun.misc.BASE64Decoder;import sun.misc.BASE64Encoder;import javax.imageio.ImageIO;import java.awt.*;import java.awt.image.BufferedImage;import java.io.*;/** * PDF文檔轉(zhuǎn)HTML文檔 * @author LXW * @date 2020/6/17 16:45 */public class PdfConvertHtmlUtil { /** * 日志對象 */ private static Logger logger = LoggerFactory.getLogger(PdfConvertHtmlUtil.class); /** * PDF文檔流轉(zhuǎn)Png * @param pdfFileInputStream * @return BufferedImage */ public static BufferedImage pdfStreamToPng(InputStream pdfFileInputStream){PDDocument doc = null;PDFRenderer renderer = null;try { doc = PDDocument.load(pdfFileInputStream); renderer = new PDFRenderer(doc); int pageCount = doc.getNumberOfPages(); BufferedImage image = null; for (int i = 0; i < pageCount; i++) {if (image != null) { image = combineBufferedImages(image, renderer.renderImageWithDPI(i, 144));}if (i == 0) { image = renderer.renderImageWithDPI(i, 144); // Windows native DPI}// BufferedImage srcImage = resize(image, 240, 240);//產(chǎn)生縮略圖 } return combineBufferedImages(image);} catch (IOException e) { e.printStackTrace();}finally { try {if(doc != null){doc.close();} } catch (IOException e) {e.printStackTrace(); }}return null; } /** *BufferedImage拼接處理,添加分割線 * @param images * @return BufferedImage */ public static BufferedImage combineBufferedImages(BufferedImage... images) {int height = 0;int width = 0;for (BufferedImage image : images) {//height += Math.max(height, image.getHeight()); height += image.getHeight(); width = image.getWidth();}BufferedImage combo = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);Graphics2D g2 = combo.createGraphics();int x = 0;int y = 0;for (BufferedImage image : images) {//int y = (height - image.getHeight()) / 2; g2.setStroke(new BasicStroke(2.0f));// 線條粗細 g2.setColor(new Color(193, 193, 193));// 線條顏色 g2.drawLine(x, y, width, y);// 線條起點及終點位置 g2.drawImage(image, x, y, null);//x += image.getWidth(); y += image.getHeight();}return combo; } /** * 通過Base64創(chuàng)建HTML文件并輸出html文件 * @param base64 * @param htmlPath html保存路徑 */ public static void createHtmlByBase64(String base64,String htmlPath) {StringBuilder stringHtml = new StringBuilder();PrintStream printStream = null;try { // 打開文件 printStream = new PrintStream(new FileOutputStream(htmlPath));} catch (FileNotFoundException e) { e.printStackTrace();}// 輸入HTML文件內(nèi)容stringHtml.append('<html><head>');stringHtml.append('<meta http-equiv='Content-Type' content='text/html; charset=UTF-8'>');stringHtml.append('<title></title>');stringHtml.append('</head>');stringHtml.append('<body + ' text-align: center;rn' + ' background-color: #C1C1C1;rn' + ''>');stringHtml.append('<img src='data:image/png;base64,' + base64 + '' />');stringHtml.append('<a name='head' style='position:absolute;top:0px;'></a>');//添加錨點用于返回首頁stringHtml.append('<a href='http://www.cgvv.com.cn/bcjs/4659.html#head'>回到首頁</a>');stringHtml.append('</body></html>');try { // 將HTML文件內(nèi)容寫入文件中 printStream.println(stringHtml.toString());} catch (Exception e) { e.printStackTrace();}finally { if(printStream != null){printStream.close();}} } /** * bufferedImage 轉(zhuǎn)為 base64編碼 * @param bufferedImage * @return */ public static String bufferedImageToBase64(BufferedImage bufferedImage) {ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();String png_base64 = '';try { ImageIO.write(bufferedImage, 'png', byteArrayOutputStream);// 寫入流中 byte[] bytes = byteArrayOutputStream.toByteArray();// 轉(zhuǎn)換成字節(jié) BASE64Encoder encoder = new BASE64Encoder(); // 轉(zhuǎn)換成base64串 刪除 rn png_base64 = encoder.encodeBuffer(bytes).trim() .replaceAll('n', '') .replaceAll('r', '');} catch (IOException e) { e.printStackTrace();}return png_base64; }}
測試Demo
public static void main(String[] args) {File file = new File('F:111FilesMySQL查詢語句大全集錦(經(jīng)典珍藏).pdf');String htmlPath = 'F:111FilesMySQL查詢語句大全集錦(經(jīng)典珍藏).html';InputStream inputStream = null;BufferedImage bufferedImage = null;try { inputStream = new FileInputStream(file); bufferedImage = pdfStreamToPng(inputStream); String base64_png = bufferedImageToBase64(bufferedImage); createHtmlByBase64(base64_png,htmlPath);} catch (FileNotFoundException e) { e.printStackTrace();}finally { try {if(inputStream != null){inputStream.close();} } catch (IOException e) {e.printStackTrace(); }} }
最終結(jié)果 轉(zhuǎn)換后文件
轉(zhuǎn)換后的文件內(nèi)容
文件預(yù)覽效果
到此這篇關(guān)于JAVA實現(xiàn)PDF轉(zhuǎn)HTML文檔的示例代碼的文章就介紹到這了,更多相關(guān)JAVA PDF轉(zhuǎn)HTML 內(nèi)容請搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持好吧啦網(wǎng)!
相關(guān)文章:
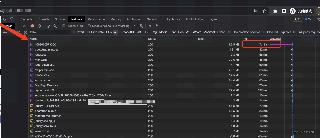